ANSI coloring and styling
Using colors in the command line tool’s output does not just look good: contrasting important elements like argument names from the rest of the text reduces the cognitive load on the user. argparse
uses ANSI escape sequences to add coloring and styling to the error messages and help text. In addition, argparse
offers public API to apply colors and styles to any text printed to the console (see below).
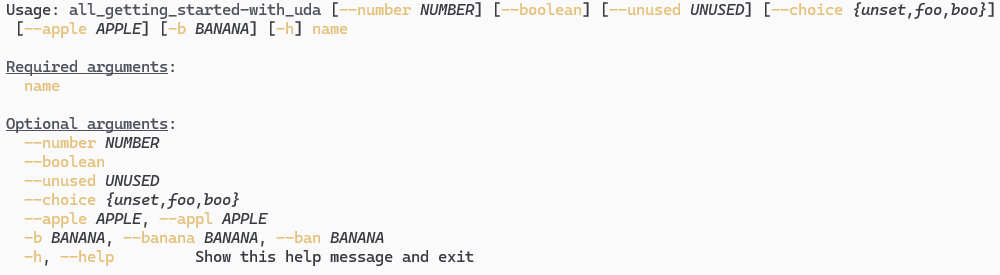
Styles and colors
The following styles and colors are available in argparse.ansi
submodule:
Font styles:
bold
italic
underline
Colors:
Foreground | Background |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
There is also a “virtual” style noStyle
that means no styling is applied. It’s useful in ternary operations as a fallback for the case when styling is disabled. See below example for details.
All styles above can be combined using .
and even be used in regular output:
The following example shows how styling can be used in custom help text (Usage
, Description
, ShortDescription
, Epilog
API):
Here is how help screen will look like:

Enable/disable the styling
By default argparse
will try to detect whether ANSI styling is supported, and if so, it will apply styling to the help text.
There is Config.stylingMode
parameter that can be used to override default behavior:
If it’s set to
Config.StylingMode.on
, then styling is always enabled.If it’s set to
Config.StylingMode.off
, then styling is always disabled.If it’s set to
Config.StylingMode.autodetect
, then heuristics are used to determine whether styling will be applied.
In some cases styling control should be exposed to a user as a command line argument (similar to --color
argument in ls
or grep
command). argparse
supports this use case – just add an argument to a command (it can be customized with @NamedArgument
UDA):
This will add the following argument:

Heuristics for enabling styling
Below is the exact sequence of steps argparse
uses to determine whether or not to emit ANSI escape codes (see detectSupport() function here for details):
If environment variable
NO_COLOR != ""
, then styling is disabled. See here for details.If environment variable
CLICOLOR_FORCE != "0"
, then styling is enabled. See here for details.If environment variable
CLICOLOR == "0"
, then styling is disabled. See here for details.If environment variable
ConEmuANSI == "OFF"
, then styling is disabled. See here for details.If environment variable
ConEmuANSI == "ON"
, then styling is enabled. See here for details.If environment variable
ANSICON
is defined (regardless of its value), then styling is enabled. See here for details.Windows only (
version(Windows)
):If environment variable
TERM
contains"cygwin"
or starts with"xterm"
, then styling is enabled.If
GetConsoleMode
call forSTD_OUTPUT_HANDLE
returns a mode that hasENABLE_VIRTUAL_TERMINAL_PROCESSING
set, then styling is enabled.If
SetConsoleMode
call forSTD_OUTPUT_HANDLE
withENABLE_VIRTUAL_TERMINAL_PROCESSING
mode was successful, then styling is enabled.
Posix only (
version(Posix)
):If
STDOUT
is not redirected, then styling is enabled.
If none of the above applies, then styling is disabled.