Config
argparse
provides decent amount of settings to customize the parser. All customizations can be done by creating Config
object with required settings (see below) and passing it to CLI API.
Assign character
Config.assignChar
is an assignment character used in arguments with value: -a=5
, -boo=foo
.
Default is equal sign =
.
Example:
Assign character
Config.assignKeyValueChar
is an assignment character used in arguments that have associative array type: -a=key=value
, -boo=key=value
.
Default is equal sign =
.
Example:
Value separator
Config.valueSep
is a separator that is used to extract argument values: -a=5,6,7
, --boo=foo,far,zoo
.
Default is ,
.
Example:
Prefix for short argument name
Config.shortNamePrefix
is a string that short names of arguments begin with.
Default is dash (-
).
Example:
Prefix for long argument name
Config.longNamePrefix
is a string that long names of arguments begin with.
Default is double dash (--
).
Example:
Variadic named arguments
Config.variadicNamedArgument
flag controls whether named arguments should be follow POSIX guidelines which allows only one value per named argument: -a value1 -a value2
.
Setting this flag to true
allows multiple values to be passed to a named argument: -a value1 value2
.
Default is false
.
Example:
End of named arguments
Config.endOfNamedArgs
is a string that marks the end of all named arguments. All arguments that are specified after this one are treated as positional regardless to the value which can start with Config.shortNamePrefix
or Config.longNamePrefix
or be a subcommand.
Default is double dash (--
).
Example:
Case sensitivity
Config
type hase three data members to allow fine-grained tuning of case sensitivity:
Config.caseSensitiveShortName
to control case sensitivity for short argument names.Config.caseSensitiveLongName
to control case sensitivity for long argument names.Config.caseSensitiveSubCommand
to control case sensitivity for subcommands.
Default value for all of them is true
.
Example:
Bundling of single-character arguments
Config.bundling
controls whether single-character arguments (usually boolean flags) can be bundled together. If it is set to true
then -abc
is the same as -a -b -c
.
Default is false
.
Example:
Adding help generation
Config.addHelpArgument
can be used to add (if true
) or not (if false
) -h
/--help
argument. In case if the command line has -h
or --help
, then the corresponding help text is printed and the parsing is stopped. If CLI!(...).parseArgs(alias newMain)
or CLI!(...).main(alias newMain)
is used, then provided newMain
function will not be called.
Default is true
.
Example:
Help text from the first part of the example code above:
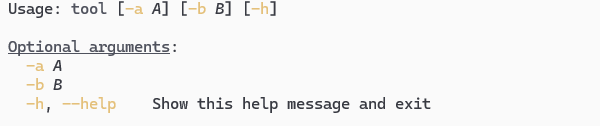
Styling mode
Config.stylingMode
controls whether styling for help text and errors should be enabled. It has the following type: enum StylingMode { autodetect, on, off }
:
Config.StylingMode.on
: styling is always enabled.Config.StylingMode.off
: styling is always disabled.Config.StylingMode.autodetect
: styling will be enabled when possible.
See ANSI coloring and styling for details.
Default value is Config.StylingMode.autodetect
.
Example:
Help text from the first part of the example code above:
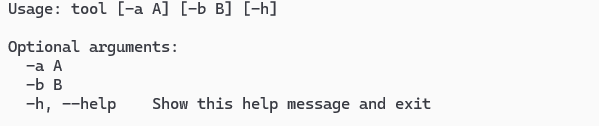
Styling scheme
Config.styling
contains style for the text output (error messages and help text). It has the following members:
programName
: style for the program name. Default isbold
.subcommandName
: style for the subcommand name. Default isbold
.argumentGroupTitle
: style for the title of argument group. Default isbold.underline
.argumentName
: style for the argument name. Default islightYellow
.namedArgumentValue
: style for the value of named argument. Default isitalic
.positionalArgumentValue
: style for the value of positional argument. Default islightYellow
.errorMessagePrefix
: style for Error: prefix in error messages. Default isred
.
See ANSI coloring and styling for details.
Example:
Help text from the first part of the example code above:
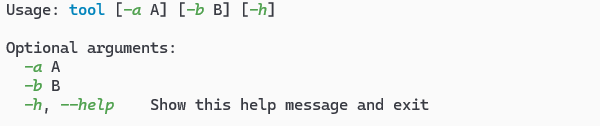
Error handling
Config.errorHandler
is a handler function for all errors occurred during command line parsing. It is a function that receives string
parameter which would contain an error message.
The default behavior is to print error message to stderr
.
Example:
This code prints Detected an error: Unrecognized arguments: ["-b"]
to stderr
.